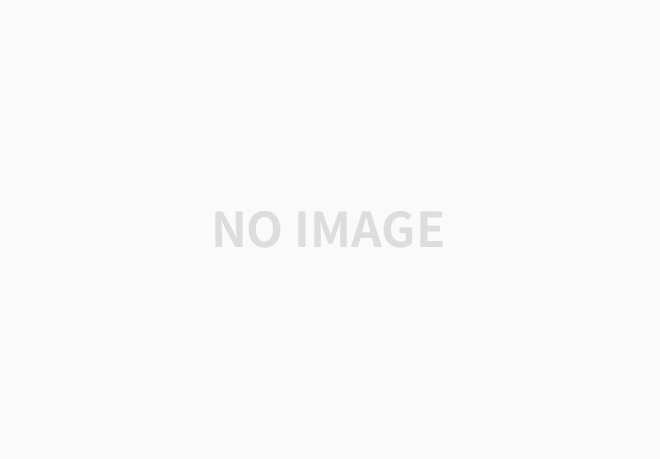
[안드로이드 스튜디오/코틀린] 커스텀 다이얼로그, 인터페이스
MainActivity.kt
package com.shinyelee.android_study2
import android.content.Intent
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.util.Log
import android.view.View
import android.widget.Button
import android.widget.Toast
// 메인화면
class MainActivity : AppCompatActivity(), MyCustomDialogInterface {
val TAG: String = "로그"
// 뷰 생성
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
// 그릴 xml 뷰 파일을 연결(설정) 시켜줌
setContentView(R.layout.activity_main)
}
fun onDialogBtnClicked(view: View) {
Log.d(TAG, "MainActivity - onDialogBtnClicked() called")
val myCustomDialog = MyCustomDialog(this, this)
myCustomDialog.show()
}
// 구독 버튼 클릭
override fun onSubscribeBtnClicked() {
Log.d(TAG, "MainActivity - onSubscribeBtnClicked() called")
Toast.makeText(this, "구독!", Toast.LENGTH_LONG).show()
}
// 좋아요 버튼 클릭
override fun onLikeBtnClicked() {
Log.d(TAG, "MainActivity - onLikeBtnClicked() called")
Toast.makeText(this, "좋아요!", Toast.LENGTH_LONG).show()
}
}
MyCustomDialog.kt
package com.shinyelee.android_study2
import android.app.Dialog
import android.content.Context
import android.graphics.Color
import android.graphics.drawable.ColorDrawable
import android.os.Bundle
import android.util.Log
import android.widget.Button
class MyCustomDialog(context: Context,
MyCustomDialogInterface: MyCustomDialogInterface)
: Dialog(context) {
val TAG: String = "로그"
private var myCustomDialogInterface: MyCustomDialogInterface? = null
// 인터페이스 연결
init {
this.myCustomDialogInterface = MyCustomDialogInterface
}
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.custom_dialog)
Log.d(TAG, "MyCustomDialog - onCreate() called")
// 배경 투명
window!!.setBackgroundDrawable(ColorDrawable(Color.TRANSPARENT))
findViewById<Button>(R.id.subscribe_btn).setOnClickListener {
Log.d(TAG, "MyCustomDialog - 구독 버튼 클릭됨")
this.myCustomDialogInterface?.onSubscribeBtnClicked()
}
findViewById<Button>(R.id.like_btn).setOnClickListener {
Log.d(TAG, "MyCustomDialog - 좋아요 버튼 클릭됨")
this.myCustomDialogInterface?.onLikeBtnClicked()
}
}
}
custom_dialog.xml
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@android:color/transparent">
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
android:layout_gravity="center"
android:gravity="center"
android:background="@drawable/rounded_corner_line"
android:padding="40dp">
<de.hdodenhof.circleimageview.CircleImageView
android:layout_width="150dp"
android:layout_height="150dp"
android:src="@drawable/paw"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="신예리의 개발자취"
android:layout_marginVertical="20dp"
android:textSize="20sp"
android:textStyle="bold"
android:textColor="#000" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="안녕하세요!\n구독!좋아요!\n부탁드립니다!!"
android:textAlignment="center"
android:lineSpacingExtra="5dp"
android:layout_marginBottom="10dp" />
<Button
android:id="@+id/subscribe_btn"
android:layout_width="250dp"
android:layout_height="wrap_content"
android:text="구독!"
android:textColor="#fff"
android:textStyle="bold"
android:layout_marginVertical="10dp"
android:background="@drawable/rounded_corner_orange" />
<Button
android:id="@+id/like_btn"
android:layout_width="250dp"
android:layout_height="wrap_content"
android:text="좋아요!"
android:textColor="#fff"
android:textStyle="bold"
android:layout_margin="10dp"
android:background="@drawable/rounded_corner_blue" />
</LinearLayout>
</FrameLayout>
MyCustomDialogInterface.kt
package com.shinyelee.android_study2
interface MyCustomDialogInterface {
fun onSubscribeBtnClicked()
fun onLikeBtnClicked()
}
메모
// 강의에서는 kotlin-android-extensions 사용
// ViewBinding으로 하면 왜인지 모르겠으나 setOnClickListener 안 먹힘
// -> 안드로이드 스튜디오 3.6 Canary 11 이상에서만 적용된다고 합
// -> 다른 프로젝트때문에 당분간 3.0 Arctic Fox 써야 함
// -> 결국 findViewById로 바꾸며 불필요한 코드 늘어남
// 버튼에 리스너 통해 행동 직접 정의하면 인터페이스 쓸 필요 없는데 굳이 쓴 이유는?
// -> 일하다보면 비슷한 상황에서 인터페이스 구현하고
// 액티비티에서 클릭이벤트 알아야 하는 경우가 있다고 함
결과물
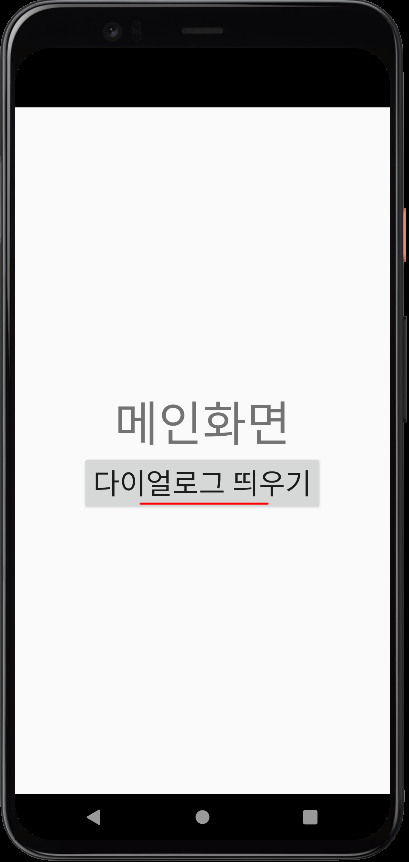
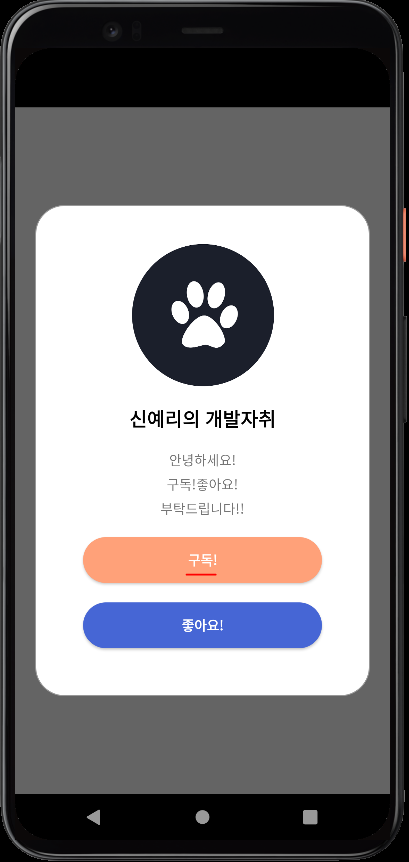
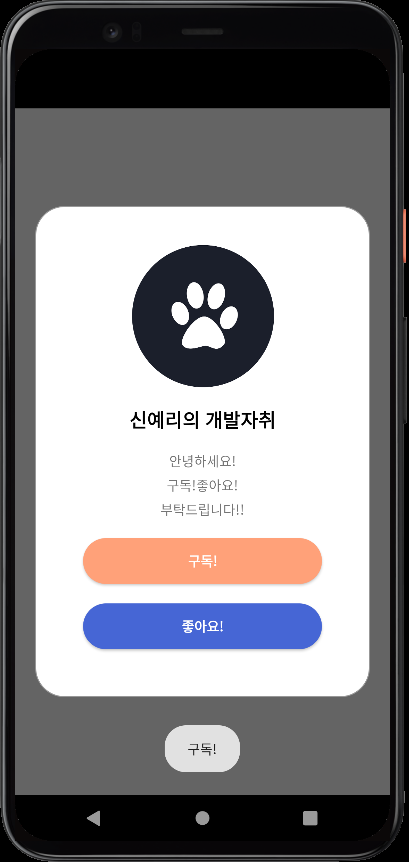
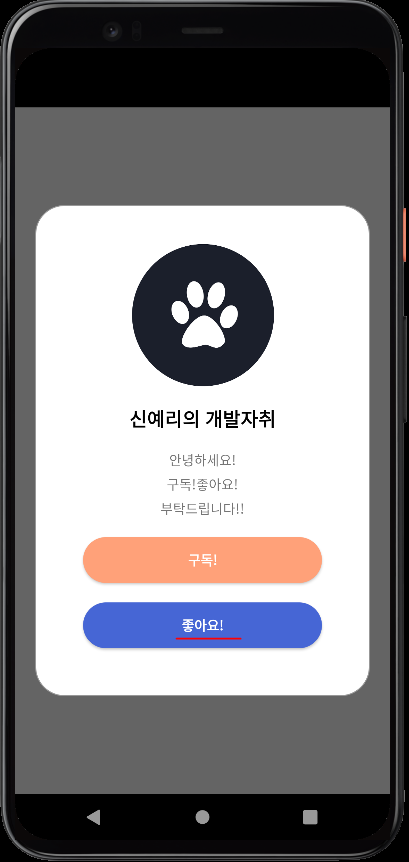
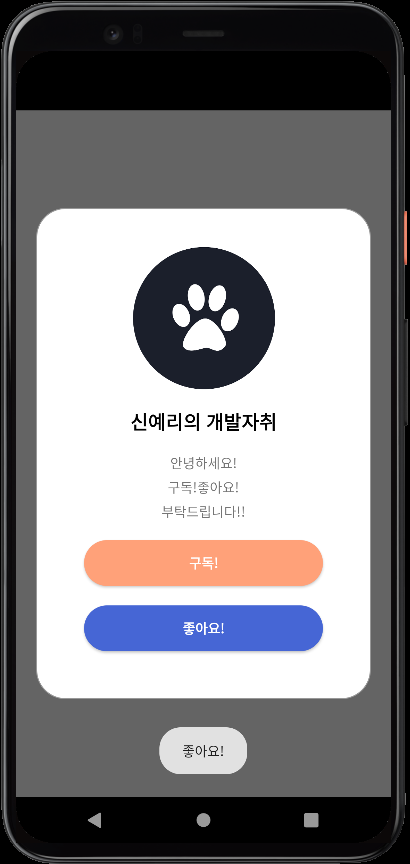
참고
반응형
'개발(Android) > android studio' 카테고리의 다른 글
[Android Studio] Font Setting (0) | 2022.04.21 |
---|---|
[Android Studio/Kotlin] Singleton Pattern (0) | 2022.04.20 |
[Android Studio/Kotlin] Listener, Method, Click Event (0) | 2022.04.18 |
[Android Studio/Kotlin] Linear Layout, Relative Layout, Constraint Layout (0) | 2022.04.17 |
[Android Studio/Kotlin] Life Cycle of Activity (0) | 2022.04.17 |